Your cart is currently empty!
TOTP authenticator PHP code [Two factor authentication] – Simple Tutorial
Here’s a simple implementation of a Time-based One-Time Password (TOTP) generator in PHP
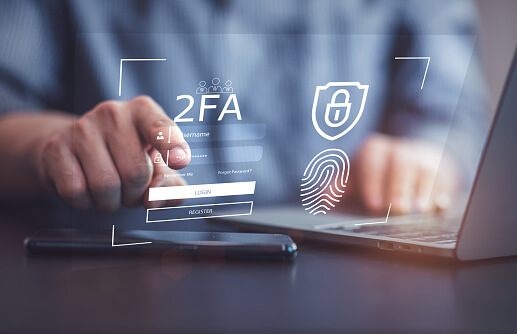
What is TOTP
TOTP is a time-based variant of the One-Time Password (OTP) authentication system. It generates a unique code that is valid only for a limited period of time (e.g., 30 seconds). This code is used to authenticate a user in the same way a password would be used.
How TOTP works
The TOTP algorithm requires two things: a secret key shared between the server and the client, and the current time.
- The client and the server agree on a secret key and a time step (e.g., 30 seconds).
- The client calculates the current time step by dividing the current time by the time step and rounding down to the nearest whole number.
- The client generates a HMAC hash of the secret key and the current time step using the SHA-1 algorithm.
- The client takes the last
n
characters of the hash, wheren
is the desired length of the TOTP code (e.g., 6 characters). - The server performs the same calculation using the secret key and the current time step and compares the result with the code received from the client.
- If the code received from the client matches the code generated by the server, the user is authenticated.
TOTP in PHP Numeric Code – Sample Example 1
<?php // Use the parameters from the latest version of the TOTP RFC (6238) $timeStep = 30; $codeLength = 6; $secret = 'JBSWY3DPEHPK3PXP'; // Compute the current time step $timestamp = floor(time() / $timeStep); // Compute the HMAC hash of the secret and the current timestamp $hash = hash_hmac('sha1', pack('N*', 0) . pack('N*', $timestamp), $secret); // Generate the TOTP code by taking the last $codeLength characters of the hash $code = substr($hash, -$codeLength); // Convert the TOTP code to a number $numericCode = 0; for ($i = 0; $i < $codeLength; $i++) { $numericCode = $numericCode * 10 + hexdec($code[$i]); } // Output the numeric TOTP echo $numericCode . PHP_EOL;
TOTP in PHP Alphanumeric Code – Sample Example 2
<?php // Use the parameters from the latest version of the TOTP RFC (6238) $timeStep = 30; $codeLength = 6; $secret = 'JBSWY3DPEHPK3PXP'; // Compute the current time step $timestamp = floor(time() / $timeStep); // Compute the HMAC hash of the secret and the current timestamp $hash = hash_hmac('sha1', pack('N*', 0) . pack('N*', $timestamp), $secret); // Generate the TOTP code by taking the last $codeLength characters of the hash $code = substr($hash, -$codeLength); // Output the TOTP echo $code;
You can simply run the PHP script in a web server or in a command-line interface (CLI) and it will output the TOTP code.
For example, if you save the script to a file called totp.php
, you can run it in the CLI with the following command:
$ php totp.php
This script generates a TOTP code that is $codeLength
characters long (in this case, 6 characters). You can use this code for authentication purposes.
Conclusion
In this tutorial, you learned how TOTP works and how to generate a TOTP code in PHP. You can use this code for authentication purposes in a variety of applications, including two-factor authentication.
Comments
Grabber Pro
Original price was: $59.$39Current price is: $39.Custom WooCommerce Checkbox Ultimate
Original price was: $39.$19Current price is: $19.Android App for Your Website
Original price was: $49.$35Current price is: $35.Abnomize Pro
Original price was: $30.$24Current price is: $24.Medical Portfolio Pro
Original price was: $31.$24Current price is: $24.
Latest Posts
- How to Create a PHP Remote File Downloader with Live Progress Bar
- How to Connect AWS CloudFront URL with a Cloudflare Subdomain
- Android Developer Interview Questions Categorized by Topic
- When Data Must be Sanitized, Escaped, and Validated in WordPress
- Alternative to WordPress for High Traffic News Websites: Node.js & Other Scalable Solutions
Leave a Reply